태그
#JQUERY
JQurey - 사용법
2021년 6월 3일 02:46
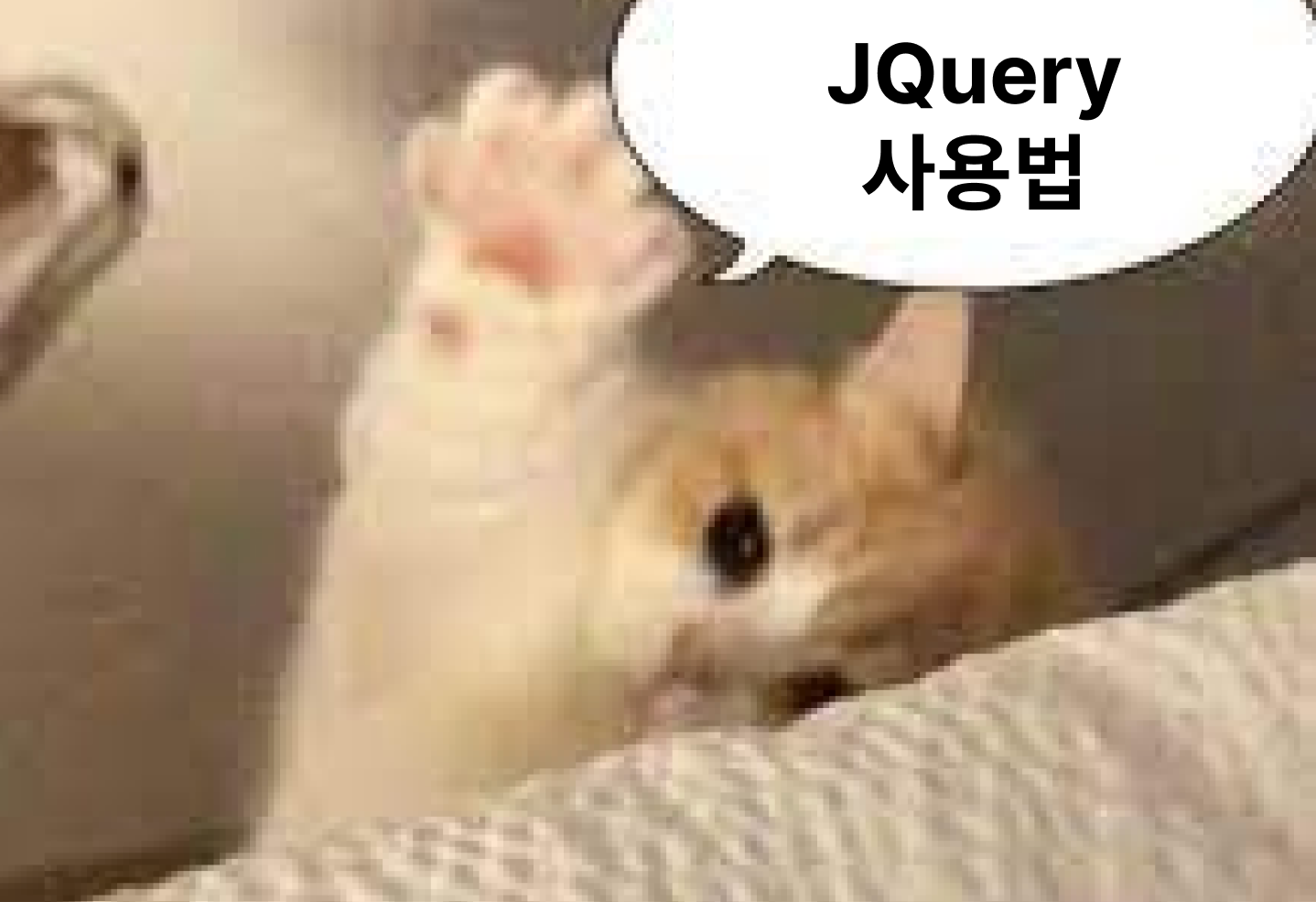
JQurey 기본 사용법
- 다운로드
- https://jquery.com/download/
- 다운로드 후 head 태그에서 include
- CMD 링크 사용
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
++ datepicker
<!-- datepicker 라이브러리 --> // jquery를 위에 써야한다 datepeicker가 jquery를 쓰기 때문
<link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
OM Tree의 Node 가져오기 (css랑 비슷)
- id명으로 node 가져오기 : $('#아이디명')
- class명으로 node 가져오기 : $('.클래스명')
- tag 명과 name 속성으로 node 가져오기 : $('태그명[name = "속성"]')
문서 로딩 이벤트
- ready : DOM Tree가 완성된 상태
- load : image나 css가 모두 load 된 상태(ready보다 이후 시점)
- error : 요소에 오류가 발생한 경우
$(document).ready(function () {
alret("DOM Tree 구성 완료");
}
마우스 동작 이벤트
- click : 요소를 클릭 했을 때
- dbclick : 요소를 더블 클릭 했을 때
- mouseover : 요소에 마우스 포인터를 올렸을 때
- mouseleave : 요소에서 마우스 포인터를 벗어났을 때
- hover : 요소에 마우스 포인터를 올렸을 때, 벗어났을 때
- scroll : 가로, 세로 스크롤바를 움직일 때
- mouseenter : 마우스 요소의 경계 외부에서 내부로 이동할 때 발생
- mouseleave : 마우스가 요소의 경계 내부에서 외부로 이동할 때 발생 9 . mousemove : 마우스를 움직일 때 발생
- mousedown : 마우스 버튼을 누를 때 발생
$(selector).on('click', function(e) {
alert("클릭 되었다");
});
키보드 이벤트
- keydown : 키를 누를 때 발생
- keypress : 글자를 입력할 때 발생
- keyup : 키로부터 손가락을 뗄 때 발생
$(selector).keyup(function() {
var inputLength = $(this).val.length;
console.log("입력된 글자 수 : " + inputLength);
}
윈도우 이벤트
- read : 문서 객체가 준비 되었을 때 발생
- load : 윈도우를 불러들일 때 발생
- unload : 윈도우를 닫을 때 발생
- resize : 윈도우의 크기를 바뀔 때 발생
- scroll : 윈도우를 스크롤 할 때 발생
- error : 에러가 있을 때 발생
$(window).load(function () {
alret("로딩 중"):
}
입력 양식 이벤트
- change : 입력 양식의 내용을 변경할 때 발생
- focus : 입력 양식에 초점을 맞추면 발생
- focusin : 입력 양식에 초점이 맞춰지기 바로 전에 발생
- blur : 입력 양식에 초점이 사라질 때 발생
- select : 입력 양식을 선택할 때 발생 (type : text, textarea 제외)
- submit : submit 버튼을 누를 때 발생
예제 )
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>jQuery</title>
<script src="https://code.jquery.com/jquery-3.6.0.js" integrity="sha256-H+K7U5CnXl1h5ywQfKtSj8PCmoN9aaq30gDh27Xc0jk=" crossorigin="anonymous"></script>
<style>
.box {width: 100px; height: 100px; background-color: aquamarine;}
</style>
<script>
$(document).ready(function() {
$('#confirmBtn').on('click', function(){
// alert("에베베벱");
// id="text"인 내용 가져오기
var text = $('#text').val();
// alert(text);
// text validation
text = text.trim();
if (text.length < 1) {
alert("텍스트 영역에 글자를 써주세요");
return;
}
// id="copyText"에 text 내용을 넣기
$('#copyText').val(text);
});
// (2) Div 안에 글자 넣기
$('textInDivBtn').on('click', function() {
//alert("버튼 클릭");
$('.box').text("박스 안에 글씨!");
});
// (3) 상자 없애기, 나타내기
$('.link1').on('click', function () {
// 상자 없애기
//$('.box').css('display', 'none');
// (1) 상자가 없으면 나타내고, 있으면 없애기
// if($('.box').css('display') == 'none') {
// $('.box').css('display', 'block');
// } else {
// $('.box').css('display', 'none');
// }
// (2) 상자가 없으면 나타내고, 있으면 없애기
if ($('#box1').is(':visible')) {
$('#box1').hide();
} else {
$('#box1').show();
}
});
// (4) 폰트 변경 클릭 이벤트
$('#fontChangeBtn').on('click', function () {
// $('#box1').addClass('box-font');
// 태그 안에 class가 이미 존재하는지 확인
if ($('box1').hasClass('box-font')) {
$('#box1').removeClass('box-font');
} else {
$('#box1').addClass('box-font');
}
});
// (5) href 속성값 변경하기
$('#gotoGoogle').on('click', function () {
//$('#gotoGoogle').attr('href', 'https://google.com');
$(this).attr('herf', "https://google.com");
});
});
</script>
</head>
<body>
텍스트 : <input type="text" id="text">
<button type="button" id="confirmBtn">확인</button><br>
복사된 텍스트 : <input type="text" id="copyText">
<div id="box1" class="box box-font"></div>
<br>
<input type="button" id="textInDivBtn" value="클락하면 박스에 글자가 들어가요!">
<br><br>
<a href="#" class="link1">박스가 사라졌다, 나타났다</a>
<br><br>
<button type="button" id="fontChangeBtn">클릭하면 상자 글씨 상태가 바뀝니다</button>
<br><br>
<a id="gotoGoogle" href="https://naver.com">여기를 누르면 구굴로 갑니다</a>
</body>
</html>